C 字符串
学习C - C字符串
字符串常量是一对双引号字符之间的字符序列。
一对双引号之间的任何内容都由编译器解释为字符串。
以下语句说明了这一点:
printf("This is a string."); printf("This is on\ntwo lines!"); printf("For \" you write \\\".");
我们可以在字符串末尾添加一个 \0
字符。
#include <stdio.h>
int main(void)
{
printf("The character \0 is used to terminate a string.");
return 0;
}
上面的代码生成以下结果。

存储字符串的变量
C没有字符串变量类型,没有处理字符串的特殊操作符。
C标准库提供一系列处理字符串的函数。
您使用一个类型为char的数组来容纳字符串。
你可以这样声明一个数组变量:
char my_string[20];
此变量可以容纳最多包含19个字符的字符串,因为必须允许一个元素作为终止字符。
您可以使用此数组来存储不是字符串的20个字符。
编译器会自动将 \0
添加到每个字符串常量的末尾。
您可以在声明它时初始化一个字符串变量:
char my_string[] = "This is a string.";
这里你没有明确定义数组维度。
编译器将为维度赋值一个值,该值足以容纳初始化字符串常量。
在这种情况下,它将是18.17个元素是字符,一个额外的元素是为终止\0。
您可以使用字符串初始化char类型的元素数组的一部分。
例如:
char str[40] = "To be";
编译器会将前五个元素str [0]初始化为str [4],其中字符串的字符为常量,str [5]将包含空字符'\ 0'。
为阵列的所有40个元素分配内存空间。
初始化char数组并将其声明为常量是处理标准消息的好方法:
const char message[] = "This is a test.";
要引用存储在数组中的字符串,请使用数组名称。
例如,要使用printf()函数输出存储在消息中的字符串,可以这样写:
printf("\nThe message is: %s", message);
%s
规范用于输出一个以null结尾的字符串。
字符串格式化
#include <stdio.h>
#define BLURB "Authentic imitation!"
int main(void)
{
printf("[%2s]\n", BLURB);
printf("[%24s]\n", BLURB);
printf("[%24.5s]\n", BLURB);
printf("[%-24.5s]\n", BLURB);
return 0;
}
上面的代码生成以下结果。
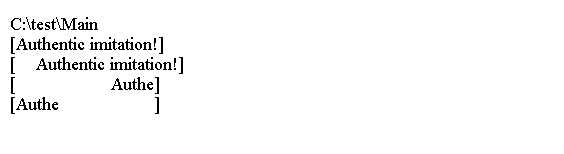
例子
以下代码显示如何获取字符串的长度。
#include <stdio.h>
int main(void) {
char str1[] = "This is a test.";
char str2[] = "This is another test.";
unsigned int count = 0; // Stores the string length
while (str1[count] != "\0") // Increment count till we reach the
++count; // terminating character.
printf("The length of the string \"%s\" is %d characters.\n", str1, count);
count = 0; // Reset count for next string
while (str2[count] != "\0") // Count characters in second string
++count;
printf("The length of the string \"%s\" is %d characters.\n", str2, count);
return 0;
}
上面的代码生成以下结果。

字符串数组
您可以存储一大堆字符串,并通过单个变量名称引用其中的任何一个。
char my_strings[3][32] = { "Hi", "Hello.", "How are you." };
虽然您必须在字符串数组中指定第二个维度,但您可以将其留给编译器以确定有多少个字符串。您可以将以前的定义写成:
char my_strings[][32] = { "Hi", "Hello.", "How are you." };
您可以使用以下代码输出三个my_strings:
for(unsigned int i = 0 ; i < sizeof(my_strings)/ sizeof(my_strings[0]) ; ++i) printf("%s\n", my_strings[i]);
以下代码显示了如何查找二维数组中的字符串数和每个字符串的长度:
#include <stdio.h>
int main(void)
{
char str[][70] = {
"hi",
"www.w3cschool.cn",
"this is a test.",
};
unsigned int count = 0; // Length of a string
unsigned int strCount = sizeof(str)/sizeof(str[0]); // Number of strings
printf("There are %u strings.\n", strCount);
// find the lengths of the strings
for(unsigned int i = 0 ; i < strCount ; ++i)
{
count = 0;
while (str[i][count])
++count;
printf("The string:\n \"%s\"\n contains %u characters.\n", str[i], count);
}
return 0;
}
上面的代码生成以下结果。
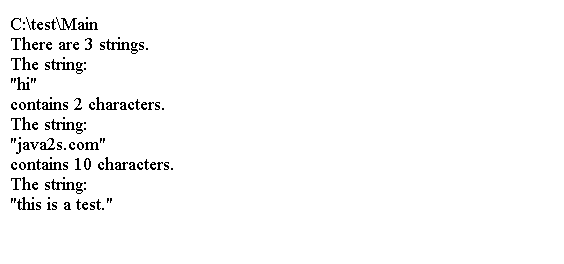
更多建议: