C 算术运算符
学习C - C算术运算符
C支持四种基本的算术运算,如加法,减法,乘法和除法。
算术语句具有以下形式:
variable_name = arithmetic_expression;
=运算符右侧的算术表达式使用存储在变量或显式数字中的值进行计算,该值使用算术运算符进行组合,如加法(+),减法( - ),乘法(*),和除法(/)。
total_count = birds + tigers + pigs + others;
下表列出了基本算术运算符。
运算符 | 操作 |
---|---|
+ | 加法 |
- | 减法 |
* | 乘法 |
/ | 除法 |
% | 求余 |
运算符应用于的值称为操作数。需要两个操作数(如%
)的运算符称为二进制运算符。
适用于单个值的运算符称为一元运算符。因此 - 是表达式a-b中的二进制运算符和表达式-data中的一元运算符。
#include <stdio.h>
int main(void)
{
int cakes = 5;
int CALORIES = 125; // Calories per cookie
int total_eaten = 0;
int eaten = 2;
cakes = cakes - eaten;
total_eaten = total_eaten + eaten;
printf("I have eaten %d cakes. There are %d cakes left", eaten, cakes);
eaten = 3; // New value for cakes eaten
cakes = cakes - eaten;
total_eaten = total_eaten + eaten;
printf("\nI have eaten %d more. Now there are %d cakes left\n", eaten, cakes);
printf("\nTotal energy consumed is %d calories.\n", total_eaten * CALORIES);
return 0;
}
上面的代码生成以下结果。
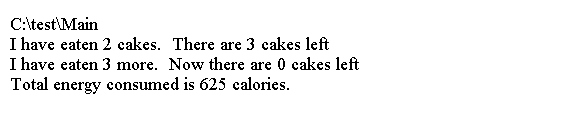
例子
以下是基本算术的代码说明:
#include <stdio.h>
int main() {
int a,b,c;
a = 10;
b = 6;
c = a + b;
printf("%d + %d = %d\n",a,b,c);
c = a - b;
printf("%d - %d = %d\n",a,b,c);
c = a * b;
printf("%d * %d = %d\n",a,b,c);
float d = a / b;
printf("%d / %d = % .2f\n",a,b,d);
float e =(float)a / b;
printf("%d / %d = % .2f\n",a,b,e);
return 0;
}
上面的代码生成以下结果。
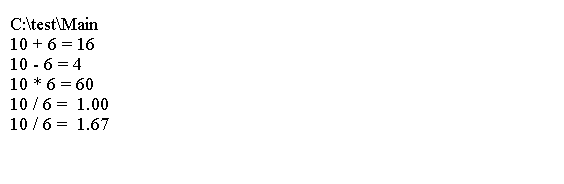
除法和求余运算符
假设你有45个蛋糕和一组七个孩子。
你会把蛋糕从小孩中分出来,并计算每个孩子的数量。
然后你会找出剩下多少蛋糕。
#include <stdio.h>
int main(void) {
int cakes = 45;
int children = 7;
int cakes_per_child = 0;
int cakes_left_over = 0;
// Calculate how many cakes each child gets when they are divided up
cakes_per_child = cakes/children;
printf("%d children and %d cakes\n", children, cakes);
printf("each child has %d cakes.\n", cakes_per_child);
cakes_left_over = cakes%children;
printf("%d cakes left over.\n", cakes_left_over);
return 0;
}
上面的代码生成以下结果。
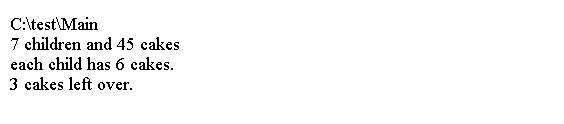
op= 赋值形式
C是一种非常简洁的语言,它为您提供了指定一些操作的缩写方式。
考虑下面的语句:
number = number + 10;
这种赋值有一个简写版本:
number += 10;
变量名后的+ =运算符是op =运算符族的一个例子。
这个语句与前一个语句的效果完全相同,它节省了一些打字。
OP中的OP可以是这些算术运算符中的任何一个:
+ - * / %
如果你假设number的值为10,你可以写下面的语句:
number *= 3; // number will be set to number*3 which is 30 number /= 3; // number will be set to number/3 which is 3 number %= 3; // number will be set to number%3 which is 1
在 op =
中的 op 也可以是一些你还没有遇到的其他操作符:
<< >> & ^ |
op =
操作符集始终以相同的方式工作。
使用 op =
的语句的一般形式:
lhs op= rhs;
其中rhs表示op =运算符右侧的任何表达式。
效果与以下语句形式相同:
lhs = lhs op (rhs);
首先,考虑这个语句:
variable *= 12;
这是相同的:
variable = variable * 12;
您现在有两种不同的方式将整数变量自增1。
以下两个语句递增计数 1:
count = count + 1; count += 1;
因为op在op =适用于计算rhs表达式的结果,语句为:
a /= b + 1;
是相同的
a = a/(b + 1);
我们所知道的除法
#include <stdio.h>
int main(void)
{
printf("integer division: 5/4 is %d \n", 5/4);
printf("integer division: 6/3 is %d \n", 6/3);
printf("integer division: 7/4 is %d \n", 7/4);
printf("floating division: 7./4. is %1.2f \n", 7./4.);
printf("mixed division: 7./4 is %1.2f \n", 7./4);
return 0;
}
上面的代码生成以下结果。
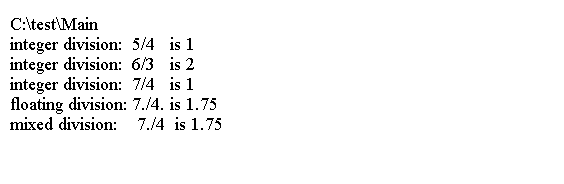
例2
以下代码显示了如何编写一个简单的计算器,当一个数字除以另一个数字时,可以添加,减法,乘法,除法和查找余数。
#include <stdio.h>
int main(void) {
double number1 = 0.0; //* First operand value a decimal number *//
double number2 = 0.0; //* Second operand value a decimal number *//
char operation = 0; //* Operation - must be +, -, *, /, or % *//
printf("\nEnter the calculation(eg. 1+2)\n");
scanf("%lf %c %lf", &number1, &operation, &number2);
switch(operation)
{
case "+": // No checks necessary for add
printf("= %lf\n", number1 + number2);
break;
case "-": // No checks necessary for subtract
printf("= %lf\n", number1 - number2);
break;
case "*": // No checks necessary for multiply
printf("= %lf\n", number1 * number2);
break;
case "/":
if(number2 == 0) // Check second operand for zero
printf("\n\n\aDivision by zero error!\n");
else
printf("= %lf\n", number1 / number2);
break;
case "%": // Check second operand for zero
if((long)number2 == 0)
printf("\n\n\aDivision by zero error!\n");
else
printf("= %ld\n", (long)number1 % (long)number2);
break;
default: // Operation is invalid if we get to here
printf("\n\n\aIllegal operation!\n");
break;
}
return 0;
}
上面的代码生成以下结果。
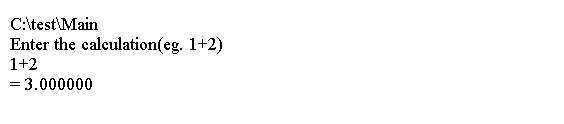
更多建议: