C for
学习C - C for
说明迭代场景的简单场景是显示数字列表。
for循环的一般模式是:
for(init_condition; control_condition ; action_per_iteration){ loop_statement; } next_statement;
要重复的语句由loop_statement表示。
init_condition
通常将一个初始值设置为一个循环控制变量。
循环控制变量跟踪循环已经完成了多少次。
您可以声明和初始化这里用逗号分隔的同一类型的几个变量。在init_condition
中定义的所有变量都是循环本地的,循环结束后不会存在。
control_condition是一个逻辑表达式,其值为true或false。
这决定循环是否应该继续执行。
只要这个条件的值为true,循环就会继续。
它通常检查循环控制变量的值。
control_condition在循环开始时进行测试,而不是结束。
如果control_condition开始为false,则loop_statement将不会被执行。
action_per_iteration在每个循环迭代结束时执行。通常是一个或多个循环控制变量的递增或递减。
在每次循环迭代时,执行loop_statement。
循环被终止,并且一旦control_condition为false,就继续执行next_statement。
以下代码显示如何将来自1的整数与用户指定的数字相加。
#include <stdio.h>
int main(void)
{
unsigned long long sum = 0LL; // Stores the sum of the integers
unsigned int count = 0; // The number of integers to be summed
// Read the number of integers to be summed
printf("\nEnter the number of integers you want to sum: ");
scanf(" %u", &count);
// Sum integers from 1 to count
for(unsigned int i = 1 ; i <= count ; ++i)
sum += i;
printf("\nTotal of the first %u numbers is %llu\n", count, sum);
return 0;
}
我们不需要在for循环语句中放置任何参数。最小的循环看起来像这样:
for( ;; ){ /* statements */ }
上面的代码生成以下结果。

例子
以下代码是进行迭代,直到数值小于10。
#include <stdio.h>
int main() {
int i;
for(i=0;i<10;i++){
printf("data %d\n",i);
}
return 0;
}
上面的代码生成以下结果。
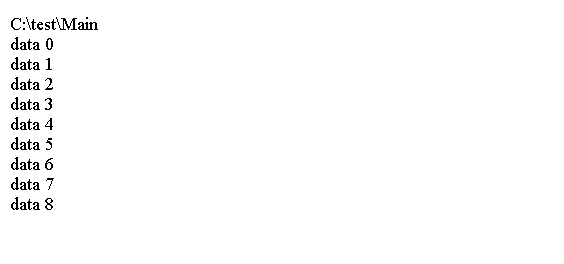
例2
您可以使用for循环执行语句多次。
要显示从1到10的数字,您可以这样写:
#include <stdio.h>
int main(void)
{
int count = 0;
for(count = 1 ; count <= 10 ; ++count)
{
printf(" %d", count);
}
printf("\nAfter the loop count has the value %d.\n", count);
return 0;
}
上面的代码生成以下结果。

例3
您不需要指定第一个控制表达式,而for循环可能如下所示:
#include <stdio.h>
int main(void)
{
int count = 1;
for( ; count <= 10 ; ++count) {
printf(" %d", count);
}
printf("\nAfter the loop count has the value %d.\n", count);
return 0;
}
上面的代码生成以下结果。

例4
下面的代码使用for循环来绘制一个盒子。
#include <stdio.h>
int main(void)
{
printf("\n**************"); // Draw the top of the box
for(int count = 1 ; count <= 8 ; ++count)
printf("\n* *"); // Draw the sides of the box
printf("\n**************\n"); // Draw the bottom of the box
return 0;
}
上面的代码生成以下结果。
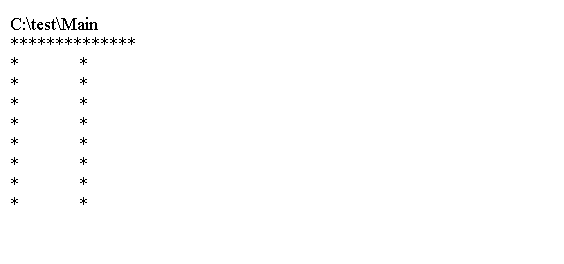
例5
这个例子演示如何在for循环中的第三个控制表达式中进行计算:
#include <stdio.h>
int main(void)
{
unsigned long long sum = 0LL; // Stores the sum of the integers
unsigned int count = 0; // The number of integers to be summed
// Read the number of integers to be summed
printf("\nEnter the number of integers you want to sum: ");
scanf(" %u", &count);
// Sum integers from 1 to count
for(unsigned int i = 1 ; i <= count ; sum += i++);
printf("\nTotal of the first %u numbers is %llu\n", count, sum);
return 0;
}
上面的代码生成以下结果。

修改for循环控制变量
以下代码显示将循环控制变量递增一定数量,并将前n个整数向后舍入。
#include <stdio.h>
int main(void)
{
unsigned long long sum = 0LL; // Stores the sum of the integers
unsigned int count = 0; // The number of integers to be summed
// Read the number of integers to be summed
printf("\nEnter the number of integers you want to sum: ");
scanf(" %u", &count);
// Sum integers from count to 1
for(unsigned int i = count ; i >= 1 ; sum += i--);
printf("\nTotal of the first %u numbers is %llu\n", count, sum);
return 0;
}
上面的代码生成以下结果。

使用for循环限制输入
您可以使用for循环来限制用户的输入量。
#include <stdio.h>
int main(void)
{
int chosen = 5; // The lucky number
int guess = 0; // Stores a guess
int count = 3; // The maximum number of tries
printf("\nGuessing game.");
for( ; count > 0 ; --count)
{
printf("\nYou have %d tr%s left.", count, count == 1 ? "y" : "ies");
printf("\nEnter a guess: "); // Prompt for a guess
scanf("%d", &guess); // Read in a guess
// Check for a correct guess
if(guess == chosen)
{
printf("\nCorrect!\n");
return 0; // End the program
}
else if(guess < 1 || guess > 10) // Check for an invalid guess
printf("between 1 and 10.\n ");
else
printf("Sorry, %d is wrong. My number is %s than that.\n",
guess, chosen > guess ? "greater" : "less");
}
printf("\nYou have had three tries and failed. The number was %d\n", chosen);
return 0;
}
上面的代码生成以下结果。
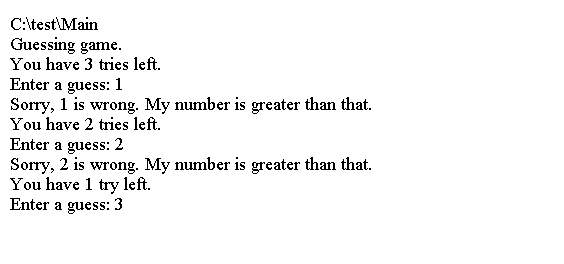
嵌套循环
你可以将一个循环放在另一个循环中。
以下代码显示如何输出给定宽度和高度的框。
#include <stdio.h>
int main(void) {
int width = 10;
int height = 30;
// Output the top of the box with width asterisks
for(unsigned int i = 0 ; i < width ; ++i)
printf("*");
// Output height-2 rows of width characters with * at each end and spaces inside
for(unsigned int j = 0 ; j < height - 2 ; ++j) {
printf("\n*"); // First asterisk
// Next draw the spaces
for(unsigned int i = 0 ; i < width - 2 ; ++i)
printf(" ");
printf("*"); // Last asterisk
}
// Output the bottom of the box
printf("\n"); // Start on newline
for(unsigned int i = 0 ; i < width ; ++i)
printf("*");
printf("\n"); // Newline at end of last line
return 0;
}
上面的代码生成以下结果。
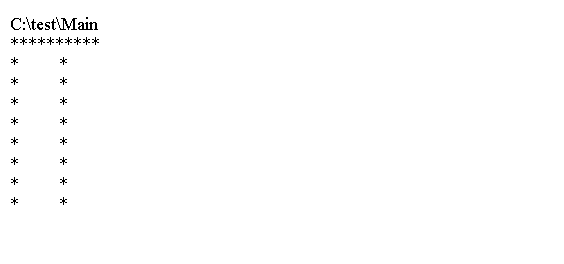
例7
以下代码使用嵌套循环来获得连续整数序列的和。
#include <stdio.h>
int main(void)
{
unsigned long sum = 0UL; // Stores the sum of integers
unsigned int count = 5; // Number of sums to be calculated
for(unsigned int i = 1 ; i <= count ; ++i)
{
sum = 0UL; // Initialize sum for the inner loop
// Calculate sum of integers from 1 to i
for(unsigned int j = 1 ; j <= i ; ++j)
sum += j;
printf("\n%u\t%5lu", i, sum); // Output sum of 1 to i
}
printf("\n");
return 0;
}
上面的代码生成以下结果。
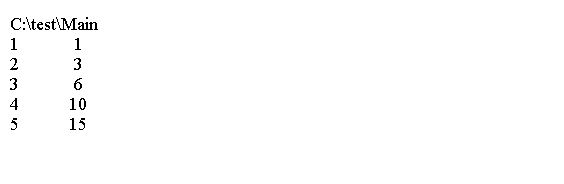
更多建议: