C 浮点类型
学习C - C浮点类型
浮点数保存用小数点写入的值。
以下是浮点值的示例:
1.6 0.00018 1234.123 100.0
最后一个常数是整数,但它将被存储为浮点值。
浮点数通常表示为十进制值乘以10的幂,其中10的幂称为指数。
下表显示了如何显示浮点数。
值 | 用C写成 |
---|---|
1.7 | 0.17E1 |
0.00009 | 0.9E-4 |
7655.123 | 0.7655123E4 |
100.0 | 1.0E2 |
/* your weight in platinum */
#include <stdio.h>
int main(void)
{
float weight; /* user weight */
float value; /* platinum equivalent */
printf("Please enter your weight in pounds: ");
/* get input from the user */
scanf("%f", &weight);
value = 3.0 * weight;
printf("Your weight is worth $%.2f.\n", value);
return 0;
}
上面的代码生成以下结果。

浮点变量
您可以选择三种类型的浮点变量,如下表所示。
关键词 | 字节数 | 值范围 |
---|---|---|
float | 4 | +/- 3.4E(+/- 38) |
double | 8 | +/-1.7E(+/-308) |
long double | 12 | +/- 1.19E(+/- 4932) |
以下代码定义了两个浮点数。
float radius; double biggest;
要写一个float类型的常量,你需要附加一个f到数字,以便将它与double类型区分开来。
当你这样声明它们时,你可以初始化前两个变量:
float radius = 2.5f; double biggest = 123E30;
可变半径的初始值为2.5,变量最大值被初始化为对应于123的数字,接着是30个零。
要指定long double常数,请附加大写或小写字母L.
long double huge = 1234567.98765L;
以下代码显示了如何浮动类型变量声明和初始化。
#include <stdio.h>
main()
{
//variable declarations
float y;
//variable initializations
y = 554.21;
//printing variable contents to standard output
printf("\nThe value of float variable y is %f", y);
}
上面的代码生成以下结果。

使用浮点值进行分割
这是一个简单的例子,它将一个浮点值除以另一个,并输出结果:
#include <stdio.h>
int main(void)
{
float length = 10.0f;
float part_count = 4.0f; // Number of equal pieces
float part_length = 0.0f;
part_length = length/part_count;
printf("%f can be divided into %f pieces and each part is f .\n", length, part_count, part_length);
return 0;
}
上面的代码生成以下结果。

小数点数
您可以在格式说明符中指定要在小数点后查看的地点数。
要将输出获得两位小数,您可以将格式说明符写为%.2f
。
要获取三位小数,您可以写入%.3f
。
以下代码更改printf()语句,以便它将产生更合适的输出:
#include <stdio.h>
int main(void)
{
float length = 10.0f;
float part_count = 4.0f; // Number of equal pieces
float part_length = 0.0f;
part_length = length/part_count;
printf("A plank %.2f feet long can be cut into %.0f pieces %.2f feet long.\n",
length, part_count, part_length);
return 0;
}
上面的代码生成以下结果。

输出字段宽度
您可以指定字段宽度和小数位数。
浮点值格式说明符的一般形式可以这样写:
%[width][.precision][modifier]f
方括号表示可选。
您可以省略宽度或精度或修饰符或这些的任何组合。
width值是一个整数,指定输出中包含空格的字符总数。它是输出字段的宽度。
precision值是指定小数点后的小数位数的整数。
当您输出的值为long double时,修饰符部分为L,否则省略。
#include <stdio.h>
int main(void)
{
float length = 10.0f;
float part_count = 4.0f; // Number of equal pieces
float part_length = 0.0f;
part_length = length/part_count;
printf("A %8.2f plank foot can be cut into %5.0f pieces %6.2f feet long.\n",
length, part_count, part_length);
const double RENT = 3852.99; // const-style constant
printf("*%f*\n", RENT);
printf("*%e*\n", RENT);
printf("*%4.2f*\n", RENT);
printf("*%3.1f*\n", RENT);
printf("*%10.3f*\n", RENT);
printf("*%10.3E*\n", RENT);
printf("*%+4.2f*\n", RENT);
printf("*%010.2f*\n", RENT);
return 0;
}
上面的代码生成以下结果。
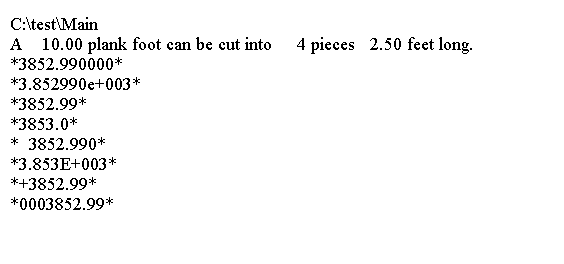
精度
要使用浮点数创建精度,请使用%符号和f字符转换说明符之间的编号方案调整转换说明符。
#include <stdio.h>
int main(void)
{
printf("%.1f", 3.123456);
printf("\n%.2f", 3.123456);
printf("\n%.3f", 3.123456);
printf("\n%.4f", 3.123456);
printf("\n%.5f", 3.123456);
printf("\n%.6f", 3.123456);
return 0;
}
上面的代码生成以下结果。
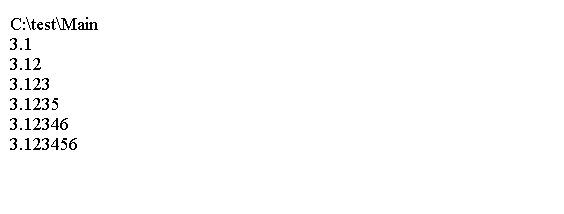
左对齐
指定字段宽度时,默认情况下,输出值将对齐。
如果您希望该值在字段中保持对齐,则只需在%之后放置一个减号。
#include <stdio.h>
int main(void)
{
float length = 10.0f;
float part_count = 4.0f; // Number of equal pieces
float part_length = 0.0f;
part_length = length/part_count;
printf("A %-18.2f plank foot can be cut into %5.0f pieces %6.2f feet long.\n",
length, part_count, part_length);
return 0;
}
您可以在字段中指定字段宽度和对齐方式,并输出整数值。
例如,%-15d指定一个整数值将被显示为左对齐的字段宽度为15个字符。
上面的代码生成以下结果。

例子
以下代码显示如何计算圆形表的圆周和面积。
#include <stdio.h>
int main(void)
{
float radius = 0.0f; // The radius of the table
float diameter = 12.12f; // The diameter of the table
float circumference = 0.0f; // The circumference of the table
float area = 0.0f; // The area of the table
float Pi = 3.14159265f;
radius = diameter/2.0f; // Calculate the radius
circumference = 2.0f*Pi*radius; // Calculate the circumference
area = Pi*radius*radius; // Calculate the area
printf("\nThe circumference is %.2f", circumference);
printf("\nThe area is %.2f\n", area);
return 0;
}
上面的代码生成以下结果。

例2
以下代码使用来自limit.h和float的定义的常量。
#include <stdio.h>
#include <limits.h> // integer limits
#include <float.h> // floating-point limits
int main(void)
{
printf("Some number limits for this system:\n");
printf("Biggest int: %d\n", INT_MAX);
printf("Smallest long long: %lld\n", LLONG_MIN);
printf("One byte = %d bits on this system.\n", CHAR_BIT);
printf("Largest double: %e\n", DBL_MAX);
printf("Smallest normal float: %e\n", FLT_MIN);
printf("float precision = %d digits\n", FLT_DIG);
printf("float epsilon = %e\n", FLT_EPSILON);
return 0;
}
上面的代码生成以下结果。
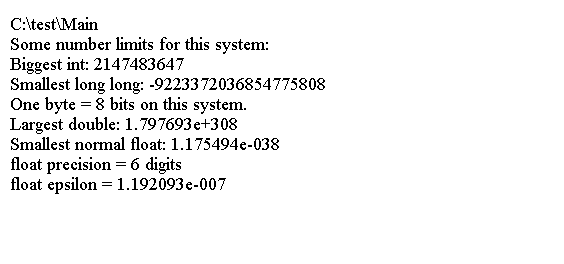
例3
以下代码以两种方式显示浮点值。
#include <stdio.h>
int main(void) {
float aboat = 32000.0;
double abet = 2.14e9;
long double dip = 5.32e-5;
printf("%f can be written %e\n", aboat, aboat);
// next line requires C99 or later compliance
printf("And it"s %a in hexadecimal, powers of 2 notation\n", aboat);
printf("%f can be written %e\n", abet, abet);
printf("%Lf can be written %Le\n", dip, dip);
return 0;
}
上面的代码生成以下结果。
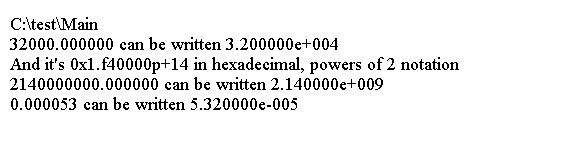
例4
读取命令行的浮点型号。
#include <stdio.h>
#include <string.h> // for strlen() prototype
#define DENSITY 62.4 // human density in lbs per cu ft
int main()
{
float weight, volume;
int size, letters;
char name[40]; // name is an array of 40 chars
printf("Hi! What"s your first name?\n");
scanf("%s", name);
printf("%s, what"s your weight in pounds?\n", name);
scanf("%f", &weight);
size = sizeof name;
letters = strlen(name);
volume = weight / DENSITY;
printf("%s, your volume is %2.2f cubic feet.\n", name, volume);
printf("first name has %d letters,\n", letters);
printf("we have %d bytes to store it.\n", size);
return 0;
}
上面的代码生成以下结果。
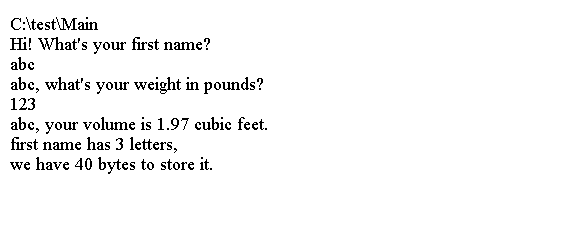
更多建议: