C++ 运算符重载
2018-03-24 15:10 更新
学习C++ - C++运算符重载
要使运算符过载,您使用一个称为运算符函数的特殊函数。
运算符函数具有以下形式,其中op是运算符被重载的符号:
operator op (argument-list)
例如,运算符+()重载+运算符,运算符*()重载*运算符。
op必须是有效的C++运算符。
下表列出了可以重载的运算符。
+ - * / % ^ & | ~ ! = < > += -= *= /= %= ^= &= |= << >> >>= <<= == != <= >= && || ++ -- , ->* -> () [] new delete new [] delete []
添加加法运算符
以下代码将Time类转换为使用重载的加法运算符。
#include <iostream>
class Time {
private:
int hours;
int minutes;
public:
Time();
Time(int h, int m = 0);
void AddMin(int m);
void AddHr(int h);
void Reset(int h = 0, int m = 0);
Time operator+(const Time & t) const;
void Show() const;
};
Time::Time()
{
hours = minutes = 0;
}
Time::Time(int h, int m )
{
hours = h;
minutes = m;
}
void Time::AddMin(int m)
{
minutes += m;
hours += minutes / 60;
minutes %= 60;
}
void Time::AddHr(int h)
{
hours += h;
}
void Time::Reset(int h, int m)
{
hours = h;
minutes = m;
}
Time Time::operator+(const Time & t) const
{
Time sum;
sum.minutes = minutes + t.minutes;
sum.hours = hours + t.hours + sum.minutes / 60;
sum.minutes %= 60;
return sum;
}
void Time::Show() const
{
std::cout << hours << " hours, " << minutes << " minutes";
}
int main()
{
using std::cout;
using std::endl;
Time planning;
Time coding(2, 40);
Time fixing(5, 55);
Time total;
cout << "planning time = ";
planning.Show();
cout << endl;
cout << "coding time = ";
coding.Show();
cout << endl;
cout << "fixing time = ";
fixing.Show();
cout << endl;
total = coding + fixing;
// operator notation
cout << "coding + fixing = ";
total.Show();
cout << endl;
Time morefixing(3, 28);
cout << "more fixing time = ";
morefixing.Show();
cout << endl;
total = morefixing.operator+(total);
// function notation
cout << "morefixing.operator+(total) = ";
total.Show();
cout << endl;
return 0;
}
上面的代码生成以下结果。
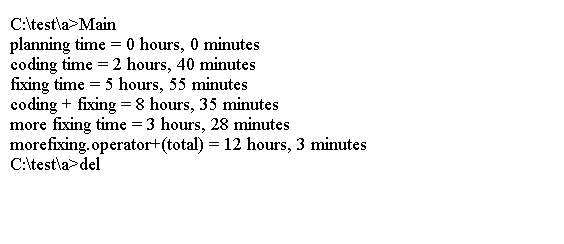
更多的重载运算符
以下代码显示了如何重载减法和乘法运算符。
#include <iostream>
class Time {
private:
int hours;
int minutes;
public:
Time();
Time(int h, int m = 0);
void AddMin(int m);
void AddHr(int h);
void Reset(int h = 0, int m = 0);
Time operator+(const Time & t) const;
Time operator-(const Time & t) const;
Time operator*(double n) const;
void Show() const;
};
Time::Time() {
hours = minutes = 0;
}
Time::Time(int h, int m )
{
hours = h;
minutes = m;
}
void Time::AddMin(int m)
{
minutes += m;
hours += minutes / 60;
minutes %= 60;
}
void Time::AddHr(int h)
{
hours += h;
}
void Time::Reset(int h, int m)
{
hours = h;
minutes = m;
}
Time Time::operator+(const Time & t) const
{
Time sum;
sum.minutes = minutes + t.minutes;
sum.hours = hours + t.hours + sum.minutes / 60;
sum.minutes %= 60;
return sum;
}
Time Time::operator-(const Time & t) const
{
Time diff;
int tot1, tot2;
tot1 = t.minutes + 60 * t.hours;
tot2 = minutes + 60 * hours;
diff.minutes = (tot2 - tot1) % 60;
diff.hours = (tot2 - tot1) / 60;
return diff;
}
Time Time::operator*(double mult) const
{
Time result;
long totalminutes = hours * mult * 60 + minutes * mult;
result.hours = totalminutes / 60;
result.minutes = totalminutes % 60;
return result;
}
void Time::Show() const
{
std::cout << hours << " hours, " << minutes << " minutes";
}
int main()
{
using std::cout;
using std::endl;
Time movie_time(4, 35);
Time dinner_time(2, 47);
Time total;
Time diff;
Time adjusted;
cout << "movie_time time = ";
movie_time.Show();
cout << endl;
cout << "dinner_time time = ";
dinner_time.Show();
cout << endl;
cout << "total work time = ";
total = movie_time + dinner_time; // use operator+()
total.Show();
cout << endl;
diff = movie_time - dinner_time; // use operator-()
cout << "movie_time time - dinner_time time = ";
diff.Show();
cout << endl;
adjusted = total * 1.5; // use operator+()
cout << "adjusted work time = ";
adjusted.Show();
cout << endl;
return 0;
}
上面的代码生成以下结果。
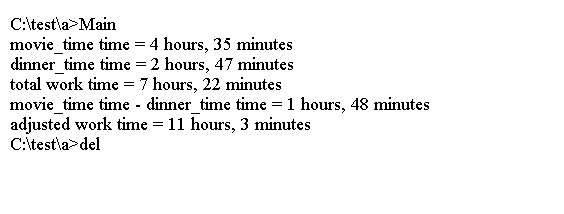
以上内容是否对您有帮助:
← C++ 构造函数
更多建议: