C++ 函数默认参数
2018-03-24 17:40 更新
学习C++ - C++函数默认参数
默认参数是一个自动使用的值,如果从函数调用中省略相应的实际参数。
例如,如果在my_func(int n)n中的默认值为1,则调用my_func()的函数与my_func(1)相同。
要建立默认值,请使用函数原型。
编译器查看原型以查看函数使用多少个参数。
以下原型使用默认参数创建一个函数。
char * left(const char * str, int n = 1);
上面的函数返回一个char指针。
要使原始字符串不变,使用第一个参数的const限定符。
您希望n的默认值为1,因此您将该值赋值给n。
默认参数值是一个初始化值。
如果你只留下n,它的值为1,但是如果你传递一个参数,新的值将覆盖1。
当您使用具有参数列表的函数时,必须从右到左添加默认值。
int my_func(int n, int m = 4, int j = 5); // VALID int my_func(int n, int m = 6, int j); // INVALID int my_func(int k = 1, int m = 2, int n = 3); // VALID
例子
// Using default arguments.
#include <iostream>
using namespace std;
// function prototype that specifies default arguments
int boxVolume( int length = 1, int width = 1, int height = 1 );
int main()
{
// no arguments--use default values for all dimensions
cout << "The default box volume is: " << boxVolume();
// specify length; default width and height
cout << "\n\nThe volume of a box with length 10,\n"
<< "width 1 and height 1 is: " << boxVolume( 10 );
// specify length and width; default height
cout << "\n\nThe volume of a box with length 10,\n"
<< "width 5 and height 1 is: " << boxVolume( 10, 5 );
// specify all arguments
cout << "\n\nThe volume of a box with length 10,\n"
<< "width 5 and height 2 is: " << boxVolume( 10, 5, 2 )
<< endl;
} // end main
// function boxVolume calculates the volume of a box
int boxVolume( int length, int width, int height )
{
return length * width * height;
}
上面的代码生成以下结果。
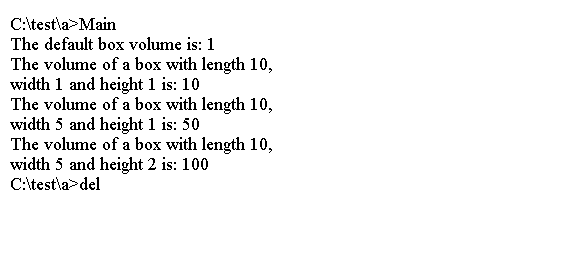
例2
以下代码创建一个带有默认参数的字符串函数。
#include <iostream>
using namespace std;
const int SIZE = 80;
char * left(const char * str, int n = 1);
int main(){
char sample[SIZE];
cout << "Enter a string:\n";
cin.get(sample,SIZE);
char *ps = left(sample, 4);
cout << ps << endl;
delete [] ps; // free old string
ps = left(sample);
cout << ps << endl;
delete [] ps; // free new string
return 0;
}
char * left(const char * str, int n){
if(n < 0)
n = 0;
char * p = new char[n+1];
int i;
for (i = 0; i < n && str[i]; i++)
p[i] = str[i]; // copy characters
while (i <= n)
p[i++] = "\0"; // set rest of string to "\0"
return p;
}
上面的代码生成以下结果。
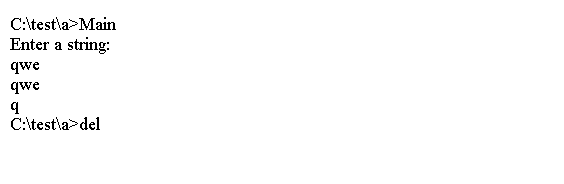
以上内容是否对您有帮助:
← C++ 函数指针
更多建议: