C++ 字符串类
2018-03-24 11:29 更新
学习C++ - C++字符串类
C++有一个字符串类。
您可以使用类型字符串变量。
要使用字符串类,程序必须包括字符串头文件。
字符串类是std命名空间的一部分。
该类可以让您将字符串视为普通变量。
例子
以下代码说明了字符串对象和字符数组之间的一些相似之处和差异。
#include <iostream>
#include <string> // make string class available
using namespace std;
int main() {
char my_char1[20]; // create an empty array
char my_char2[20] = "C++"; // create an initialized array
string str1; // create an empty string object
string str2 = "Java"; // create an initialized string
cout << "Enter a string: ";
cin >> my_char1;
cout << "Enter another string: ";
cin >> str1; // use cin for input
cout << my_char1 << " " << my_char2 << " "
<< str1 << " " << str2 // use cout for output
<< endl;
cout << "The third letter in " << my_char2 << " is "
<< my_char2[2] << endl;
cout << "The third letter in " << str2 << " is "
<< str2[2] << endl; // use array notation
return 0;
}
上面的代码生成以下结果。
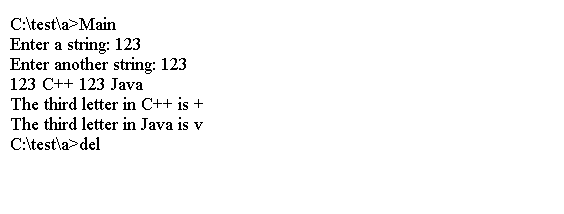
例2
以下代码显示了一些String用法。
请注意,您可以将C风格的字符串以及字符串对象添加到字符串对象。
#include <iostream>
#include <string> // make string class available
using namespace std;
int main() {
string s1 = "www.w3cschool.cn";
string s2, s3;
cout << "You can assign one string object to another: s2 = s1\n";
s2 = s1;
cout << "s1: " << s1 << ", s2: " << s2 << endl;
cout << "You can assign a C-style string to a string object.\n";
cout << "s2 = \"new Value\"\n";
s2 = "C++";
cout << "s2: " << s2 << endl;
cout << "You can concatenate strings: s3 = s1 + s2\n";
s3 = s1 + s2;
cout << "s3: " << s3 << endl;
cout << "You can append strings.\n";
s1 += s2;
cout <<"s1 += s2 yields s1 = " << s1 << endl;
s2 += " for a day";
cout <<"s2 += \" for a day\" yields s2 = " << s2 << endl;
return 0;
}
上面的代码生成以下结果。
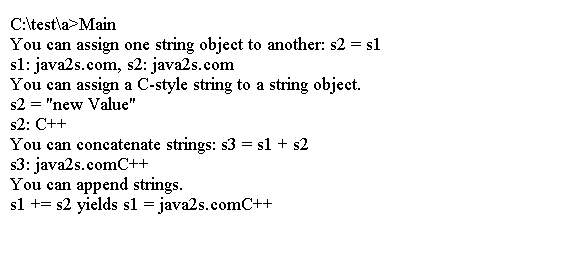
例3
C库相当于
str3 = str1 + str2;
这是:
strcpy(my_char3, my_char1); strcat(my_char3, my_char2);
以下代码将使用字符串对象的技术与使用字符数组的技术进行比较。
#include <iostream>
#include <string> // make string class available
#include <cstring> // C-style string library
using namespace std;
int main() {
char my_char1[20];
char my_char2[20] = "www.w3cschool.cn";
string str1;
string str2 = "C++";
str1 = str2; // copy str2 to str1
strcpy(my_char1, my_char2); // copy my_char2 to my_char1
str1 += " vs "; // add to the end of str1
strcat(my_char1, " Java"); // add to the end of my_char1
int len1 = str1.size(); // obtain length of str1
int len2 = strlen(my_char1);// obtain length of my_char1
cout << "The string " << str1 << " contains "
<< len1 << " characters.\n";
cout << "The string " << my_char1 << " contains "
<< len2 << " characters.\n";
return 0;
}
上面的代码生成以下结果。
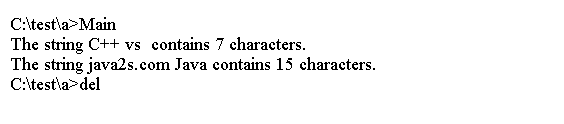
字符串类I/O
您可以使用cin与>>操作符来读取一个字符串对象,并使用<<操作符来显示一个使用与C风格字符串相同的语法的字符串对象。
一次读取一行而不是一个字使用不同的语法。
#include <iostream>
#include <string> // make string class available
#include <cstring> // C-style string library
using namespace std;
int main() {
char my_char[20];
string str;
cout << "Length of string: "
<< strlen(my_char) << endl;
cout << "Length of string in str before input: "
<< str.size() << endl;
cout << "Enter a line of text:\n";
cin.getline(my_char, 20); // indicate maximum length
cout << "You entered: " << my_char << endl;
cout << "Enter another line of text:\n";
getline(cin, str); // cin now an argument; no length specifier
cout << "You entered: " << str << endl;
cout << "Length of string in my_char after input: "
<< strlen(my_char) << endl;
cout << "Length of string in str after input: "
<< str.size() << endl;
return 0;
}
上面的代码生成以下结果。
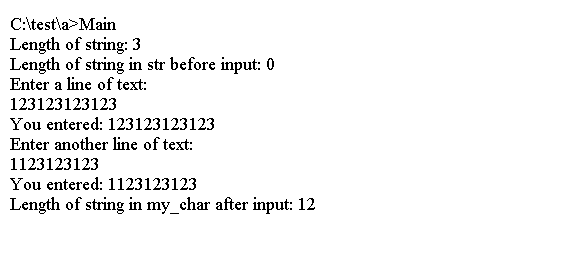
以上内容是否对您有帮助:
← C++ 类型转换
更多建议: