C++ 整数类型
学习C++ - C++整数类型
整数是没有小数部分的数字,例如2,98,?5286和0。
各种C ++整数类型在保存整数时使用的内存量不同。
某些类型(有符号类型)可以表示正值和负值,而其他类型(无符号类型)不能表示负值。
用于描述用于整数的内存量的通常术语是width。
C ++的基本整数类型,按照增加宽度的顺序,是char,short,int,long,和C ++ 11,long long。
每个都有签名和无符号版本。
这样可以选择十种不同的整数类型!
short,int,long和long long整数类型。
通过使用不同数量的位来存储值,C ++类型short,int,long和long long可以表示最多四个不同的整数宽度。
短整数至少为16位宽。
int整数至少与short一样大。
长整数至少为32位宽,至少与int一样大。
长整数长至少64位宽,至少长达一个大。
您可以使用这些类型的名称来声明变量,就像使用int一样:
short score; // creates a type short integer variable int temperature; // creates a type int integer variable long position; // creates a type long integer variable
如果您想知道系统的整数大小,您可以使用C ++工具来调查程序的类型大小。
sizeof运算符返回类型或变量的大小(以字节为单位)。
// Writing values of variables to cout
#include <iostream>
int main()
{
int apple_count {15}; // Number of apples
int orange_count {5}; // Number of oranges
int total_fruit {apple_count + orange_count}; // Total number of fruit
std::cout << "The value of apple_count is " << apple_count << std::endl;
std::cout << "The value of orange_count is " << orange_count << std::endl;
std::cout << "The value of total_fruit is " << total_fruit << std::endl;
}
上面的代码生成以下结果。
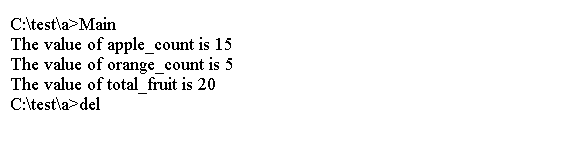
带符号的整数类型
下表显示了存储带符号整数的完整的基本类型集,即正值和负值。
为每种类型分配的内存,因此可以存储的值的范围可能在不同的编译器之间变化。
类型名称 | 典型尺寸(字节) | 值范围 |
---|---|---|
signed char | 1 | -128〜127 |
short/short int | 2 | -256〜255 |
int | 4 | -2,147,483,648至+2,147,483,647 |
long/long int | 4 | -2,147,483,648至+2,147,483,647 |
long long/long long int | 8 | -9,223,372,036,854,775,808至9,223,372,036,854,775,807 |
例子
以下代码显示如何获取一些整数限制。
#include <iostream>
#include <climits> // use limits.h for older systems
int main() {
using namespace std;
int n_int = INT_MAX; // initialize n_int to max int value
short n_short = SHRT_MAX; // symbols defined in climits file
long n_long = LONG_MAX;
long long n_llong = LLONG_MAX;
// sizeof operator yields size of type or of variable
cout << "int is " << sizeof (int) << " bytes." << endl;
cout << "short is " << sizeof n_short << " bytes." << endl;
cout << "long is " << sizeof n_long << " bytes." << endl;
cout << "long long is " << sizeof n_llong << " bytes." << endl;
cout << endl;
cout << "Maximum values:" << endl;
cout << "int: " << n_int << endl;
cout << "short: " << n_short << endl;
cout << "long: " << n_long << endl;
cout << "long long: " << n_llong << endl << endl;
cout << "Minimum int value = " << INT_MIN << endl;
cout << "Bits per byte = " << CHAR_BIT << endl;
return 0;
}
上面的代码生成以下结果。
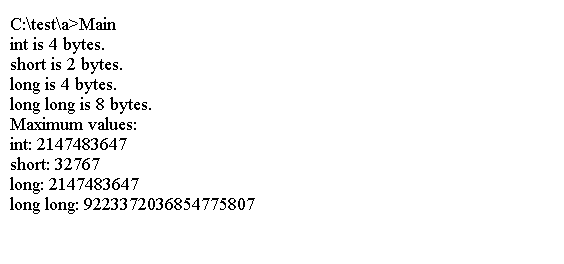
注意
下表列出了来自climits的符号常数。
符号常数 | 表示 |
---|---|
CHAR_BIT | 字符中的位数 |
CHAR_MAX | 最大字符值 |
CHAR_MIN | 最小字符值 |
SCHAR_MAX | 最大签名字符值 |
SCHAR_MIN | 最小签名字符值 |
UCHAR_MAX | 最大无符号字符值 |
SHRT_MAX | 最大短值 |
SHRT_MIN | 最小短值 |
USHRT_MAX | 最大无符号短整型值 |
INT_MAX | 最大int值 |
INT_MIN | 最小int值 |
UINT_MAX | 最大无符号整数值 |
LONG_MAX | 最大长整数值 |
LONG_MIN | 最小长值 |
ULONG_MAX | 最大无符号长整型值 |
LLONG_MAX | 最大长整型值 |
LLONG_MIN | 最小长整型值 |
ULLONG_MAX | 最大unsigned long long值 |
初始化
初始化将赋值与声明组合在一起。
例如,以下语句声明n_int变量并将其设置为可能的最大类型int值:
int n_int = INT_MAX;
您也可以使用文字常量(如255)来初始化值。
您可以将变量初始化为表达式,前提是程序执行到达声明时表达式中的所有值都已知:
int uncles = 5; // initialize uncles to 5 int aunts = uncles; // initialize aunts to 5 int chairs = aunts + uncles + 4; // initialize chairs to 14
无符号类型
您学习的四个整数类型中的每一个都具有无法保存负值的无符号变量。
这具有增加变量可以容纳的最大值的优点。
如果short表示范围为32,768到+32,767,则无符号版本可以表示范围0到65,535。
对于永不为负的值,您应该使用无符号类型,例如人口。
要创建基本整数类型的无符号版本,您只需使用unsigned关键字即可修改声明:
unsigned short change; // unsigned short type unsigned int rovert; // unsigned int type unsigned quarterback; // also unsigned int unsigned long gone; // unsigned long type unsigned long long lang_lang; // unsigned long long type
unsigned是unsigned int的缩写。
以下代码说明了使用无符号类型。
它还显示如果您的程序尝试超出整数类型的限制,可能会发生什么。
#include <iostream>
#include <climits> // defines INT_MAX as largest int value
#define ZERO 0 // makes ZERO symbol for 0 value
int main()
{
using namespace std;
short s = SHRT_MAX; // initialize a variable to max value
unsigned short sue = s;
cout << "s has " << s << " dollars and Sue has " << sue;
cout << "Add $1 to each account." << endl << "Now ";
s = s + 1;
sue = sue + 1;
cout << "s has " << s << " dollars and Sue has " << sue;
s = ZERO;
sue = ZERO;
cout << "s has " << s << " dollars and Sue has " << sue;
s = s - 1;
sue = sue - 1;
cout << "s has " << s << " dollars and Sue has " << sue;
cout << "Hi!" << endl;
return 0;
}
上面的代码生成以下结果。
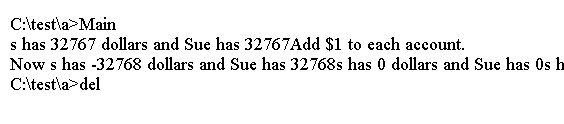
整数文字
整数文字或常数是您明确写出的整数,例如212或17。
C++,像C一样,可以在三个不同的数字基础中写入整数:base 10,base 8和base 16。
C++使用第一位或第二位来标识数字常量的基数。
如果第一位数字在1〜9范围内,则为10位(十进制);因此93是10。
如果第一位为0,第二位为1〜7,则为8位(八进制);因此042是八进制,等于34进制数。
如果前两个字符为0x或0X,则该数字为16位(十六进制);因此0x42是十六进制,等于66十进制。
对于十六进制值,字符a?f和A?F表示对应于值10〜15的十六进制数字。
这里有一些十六进制文字的例子:
Hexadecimal literals:0x1AF 0x123U 0xAL 0xcad 0xFF Decimal literals: 431 291U 10L 3245 255
下面是八进制文字的一些例子。
Octal literals: 0657 0443U 012L 06255 0377 Decimal literals: 431 291U 10L 3245 255
0xF为15,0xA5为165(10六进制加5个)。以下代码显示了十六进制和八进制文字。
#include <iostream>
int main()
{
using namespace std;
int my_decimal = 42; // decimal integer literal
int my_hexi = 0x42; // hexadecimal integer literal
int my_oct = 042; // octal integer literal
cout << "my_decimal = " << my_decimal << " (42 in decimal)\n";
cout << "my_hexi = " << my_hexi << " (0x42 in hex)\n";
cout << "my_oct = " << my_oct << " (042 in octal)\n";
return 0;
}
上面的代码生成以下结果。
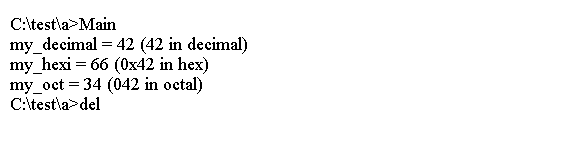
二进制文字
你写一个二进制整数字面值作为前缀为0b或0B的二进制数字(0或1)的序列。
二进制文字可以使用L或LL作为后缀来表示它是long或long long类型,如果是无符号文字则为u或U。
例如:
Binary literals: 0B110101111 0b100100011U 0b1010L 0B11001101 0b11111111 Decimal literals: 431 291U 10L 3245 255
例2
以下代码显示如何以十六进制和八进制显示值。
#include <iostream>
using namespace std;
int main()
{
int my_decimal = 42;
int my_hexi = 42;
int my_oct = 42;
cout << "my_decimal = " << my_decimal << " (decimal for 42)" << endl;
cout << hex; // manipulator for changing number base
cout << "my_hexi = " << my_hexi << " (hexadecimal for 42)" << endl;
cout << oct; // manipulator for changing number base
cout << "my_oct = " << my_oct << " (octal for 42)" << endl;
return 0;
}
上面的代码生成以下结果。
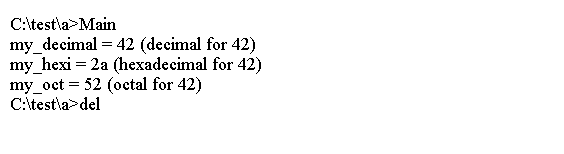
例3
转换距离
#include <iostream> // For output to the screen
int main()
{
unsigned int yards =10, feet =10, inches =10;
const unsigned int feet_per_yard {3U};
const unsigned int inches_per_foot {12U};
unsigned int total_inches {};
total_inches = inches + inches_per_foot*(yards*feet_per_yard + feet);
std::cout << "The distances corresponds to " << total_inches << " inches.\n";
std::cout << "Enter a distance in inches: ";
std::cin >> total_inches;
feet = total_inches/inches_per_foot;
inches = total_inches%inches_per_foot;
yards = feet/feet_per_yard;
feet = feet%feet_per_yard;
std::cout << "The distances corresponds to "
<< yards << " yards "
<< feet << " feet "
<< inches << " inches." << std::endl;
}
上面的代码生成以下结果。
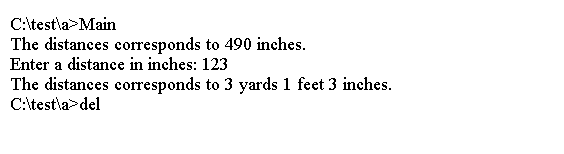
更多建议: