C++ 逻辑运算符
学习C++ - C++逻辑运算符
运算符是逻辑或,写成||;逻辑与,写&&和逻辑非,写成!。
逻辑或运算符:||
OR可以指示两种条件之一或两者满足要求。
逻辑或运算符,写入||,将两个表达式组合成一个。
如果一个或两个原件表达式为true或非零,则生成的表达式的值为true。
否则,表达式的值为false。
这里有些例子:
5 == 5 || 5 == 9 // true because first expression is true 5 > 3 || 5 > 10 // true because first expression is true 5 > 8 || 5 < 10 // true because second expression is true 5 < 8 || 5 > 2 // true because both expressions are true 5 > 8 || 5 < 2 // false because both expressions are false
因为||具有比关系运算符低的优先级,您不需要在这些表达式中使用括号。
下表总结了||运算符的工作原理。
expr1的值|| expr2
expr1 == true expr1 == false expr2 == true true true expr2 == false true false
C ++||运算符支持快捷方式评估。
例如,考虑以下表达式:
i++ < 6 || i == j
假设 i 最初的值为10。
如果表达式i ++<6是真的,C++将不会麻烦评估表达式 i== j,因为它只需要一个真实的表达式来使整个逻辑表达式为真。
例子
以下代码在if语句中使用||运算符来检查字符的大写和小写版本。
#include <iostream>
using namespace std;
int main()
{
cout << "Do you wish to continue? <y/n> ";
char ch;
cin >> ch;
if (ch == "y" || ch == "Y") // y or Y
cout << "You were warned!\a\a\n";
else if (ch == "n" || ch == "N") // n or N
cout << "A wise choice ... bye\n";
else
cout << "else";
return 0;
}
上面的代码生成以下结果。
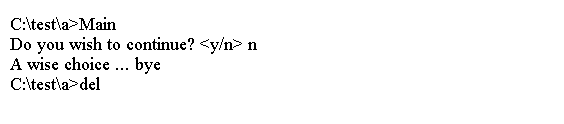
逻辑与运算符:&&
逻辑与运算符&&将两个表达式组合成一个。
如果两个原始表达式都为true,则生成的表达式的值为true。
这里有些例子:
5 == 5 && 4 == 4 // true because both expressions are true 5 > 3 && 5 > 10 // false because second expression is false
下表总结了&&运算符的工作原理。
expr1 && expr2的值
expr1 == true expr1 == false expr2 == true true false expr2 == false false false
例2
以下代码显示如何使用&&
#include <iostream>
const int SIZE = 6;
using namespace std;
int main()
{
float naaq[SIZE];
int i = 0;
float temp;
cout << "First value: ";
cin >> temp;
while (i < SIZE && temp >= 0) // 2 quitting criteria
{
naaq[i] = temp;
++i;
if (i < SIZE) // room left in the array,
{
cout << "Next value: ";
cin >> temp; // so get next value
}
}
return 0;
}
上面的代码生成以下结果。
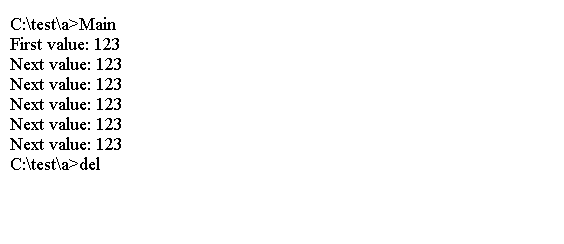
逻辑非运算符:!
!运算符否定后面的表达式的真值。
如果expression为true,则!expressionis为false,反之亦然。
以下代码显示如何使用not运算符。
#include <iostream>
#include <climits>
using namespace std;
bool is_int(double);
int main()
{
double num;
cout << "Enter an integer value: ";
cin >> num;
while (!is_int(num)) // continue while num is not int-able
{
cout << "Out of range -- please try again: ";
cin >> num;
}
int val = int (num); // type cast
cout << "You"ve entered the integer " << val << "\nBye\n";
return 0;
}
bool is_int(double x)
{
if (x <= INT_MAX && x >= INT_MIN) // use climits values
return true;
else
return false;
}
上面的代码生成以下结果。
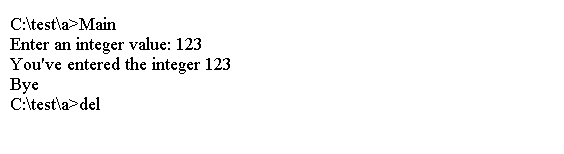
字符函数
下面的代码演示了cctype系列的一些函数。
#include <iostream>
#include <cctype> // prototypes for character functions
int main(){
using namespace std;
cout << "Enter text for analysis, and type @ to terminate input.\n";
char ch;
int whitespace = 0;
int digits = 0;
int chars = 0;
int punct = 0;
int others = 0;
cin.get(ch); // get first character
while (ch != "@") // test for sentinel
{
if(isalpha(ch)) // is it an alphabetic character?
chars++;
else if(isspace(ch)) // is it a whitespace character?
whitespace++;
else if(isdigit(ch)) // is it a digit?
digits++;
else if(ispunct(ch)) // is it punctuation?
punct++;
else
others++;
cin.get(ch); // get next character
}
cout << chars << " letters, "
<< whitespace << " whitespace, "
<< digits << " digits, "
<< punct << " punctuations, "
<< others << " others.\n";
return 0;
}
上面的代码生成以下结果。
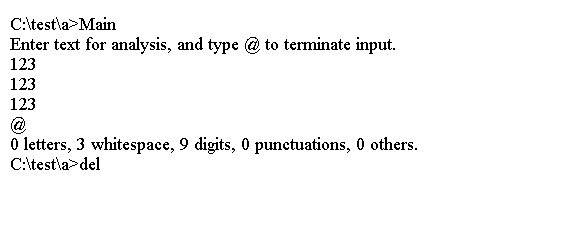
测试字符的函数
下表总结了cctype包中提供的函数。
函数名称 | 返回值 |
---|---|
isalnum() | 如果参数是字母数字(即字母或数字),则返回true。 |
isalpha() | 如果参数是字母,则返回true。 |
isblank() | 如果参数是空格或水平制表符,则返回true。 |
iscntrl() | 如果参数是控制字符,则返回true。 |
isdigit() | 如果参数是十进制数字(0-9),则返回true。 |
isgraph() | 如果参数是除空格之外的任何打印字符,则返回true。 |
islower() | 如果参数是小写字母,则返回true。 |
isprint() | 如果参数是任何打印字符,则返回true,包括空格。 |
ispunct() | 如果参数是标点符号,则返回true。 |
isspace() | 如果参数是标准空格字符(即,空格,换页,换行,回车,水平制表符,垂直制表符),则返回true。 |
isupper() | 如果参数是大写字母,则返回true。 |
isxdigit() | 如果参数是十六进制数字字符(即,0-9,a-f或A-F),则返回true。 |
tolower() | 如果参数是大写字符,tolower()返回该字符的小写版本;否则,它返回参数不变。 |
toupper() | 如果参数是小写字符,则toupper()返回该字符的大写版本;否则,它返回参数不变。 |
更多建议: