Node.js 异步编程
2018-01-13 15:07 更新
下面的代码显示了Node.js如何处理非阻塞的异步模型。
setTimeout()函数接受调用一个函数,并在超时之后应该调用它:
setTimeout(function () {
console.log("done");
}, 2000);
console.log("waiting");
如果运行上述代码,你将看到以下输出:

程序将超时设置为2000ms(2s),然后继续执行,打印出“waiting"文本。
在Node.js中,调用一个函数需要等待一些外部资源,我们应该调用fopen(path,mode,function callback(file_handle){...}),而不是调用fopen(path,mode)和waiting。
例子
下面的代码为异步函数。
var fs = require("fs");
/*www.w3cschool.cn*/
fs.open(
"a.js", "r",
function (err, handle) {
var buf = new Buffer(100000);
fs.read(
handle, buf, 0, 100000, null,
function (err, length) {
console.log(buf.toString("utf8", 0, length));
fs.close(handle, function () { /* don"t care */ });
}
);
}
);
上面的代码生成以下结果。
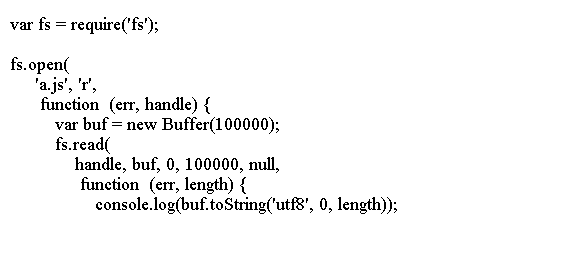
注意
require函数是一种在Node.js程序中包含附加功能的方法。
回调异步函数有至少一个参数,最后操作的成功或失败状态。它通常具有第二参数,其具有来自最后操作的附加结果或信息。
do_something(param1, param2, ..., paramN, function (err, results) { ... });
例如,
fs.open(//www.w3cschool.cn
"a.js", "r",
function (err, handle) {
if (err) {
console.log("ERROR: " + err.code + " (" + err.message ")");
return;
}
// success!! continue working here
}
);
在此样式中,你检查错误或继续处理结果。
现在这里是另一种方式:
fs.open(//www.w3cschool.cn
"a.hs", "r",
function (err, handle) {
if (err) {
console.log("ERROR: " + err.code + " (" + err.message ")");
} else {
// success! continue working here
}
}
);
以下代码显示如何读取带有错误处理程序的文件。
var fs = require("fs");
//www.w3cschool.cn
fs.open(
"a.js", "r",
function (err, handle) {
if (err) {
console.log("ERROR: " + err.code + " (" + err.message + ")");
return;
}
var buf = new Buffer(100000);
fs.read(
handle, buf, 0, 100000, null, function (err, length) {
if (err) {
console.log("ERROR: " + err.code + " (" + err.message + ")");
return;
}
console.log(buf.toString("utf8", 0, length));
fs.close(handle, function () { /* don"t care */ });
}
);
}
);
上面的代码生成以下结果。
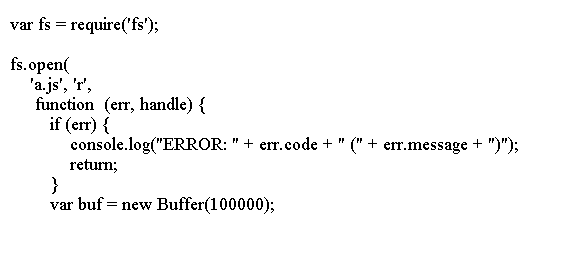
以上内容是否对您有帮助:
更多建议: