C malloc
学习C - C malloc
你也可以显式地在运行时分配内存。
你也可以显式地在运行时分配内存。...
当你使用 malloc()
函数,指定内存的字节数你想要分配作为参数。
该函数返回它的内存的第一个字节的地址分配响应您的请求。
动态内存分配的一个典型示例可能是:
int *pNumber = (int*)malloc(100);
这里,您请求了100字节的内存,并将此内存块的地址分配给pNumber。
以下代码分配内存以容纳25个整数。
int *pNumber = (int*)malloc(25*sizeof(int));
(int *)
将函数返回的地址转换为int类型的指针。
#include <stdio.h>
#include <stdlib.h>
main()
{
char *name;
name = (char *) malloc(80*sizeof(char));
if ( name != NULL ) {
printf("\nEnter your name: ");
gets(name);
printf("\nHi %s\n", name);
free(name); //free memory resources
} // end if
} //end main
上面的代码生成以下结果。

免费和malloc
下面的代码显示了处理内存分配失败的模式。
int *pNumber = (int*)malloc(25*sizeof(int)); if(!pNumber) { // Code to deal with memory allocation failure . . . }
释放动态分配的内存
当动态分配内存时,您需要在不再需要时释放内存。
要释放内存,只需写下这条语句:
free(pNumber); pNumber = NULL;
free()
函数具有类型为 void *
的形式参数,你可以传递任何类型的指针作为参数。
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
int main(void)
{
unsigned long long *pPrimes = NULL; // Pointer to primes storage area
int total = 5; // Number of primes required
// Allocate sufficient memory to store the number of primes required
pPrimes = (unsigned long long*)malloc(total*sizeof(unsigned long long));
if(!pPrimes){
printf("Not enough memory.\n");
return 1;
}
free(pPrimes); // Release the heap memory . . .
pPrimes = NULL; // . . . and reset the pointer
return 0;
}
上面的代码生成以下结果。

例子
在本节中,我们将使用指针创建一个动态数组。
一般来说,我们可以声明一个数组使用[]和size。 现在我们可以声明动态大小的数组。
基本上,当我们添加一个新值时,我们分配内存,然后将其附加到数组。
#include <stdio.h>
#include <stdlib.h>
int main(int argc, const char* argv[]) {
// define dynamic array of pointer
int *numbers; // single array pointer
// a number of array
int N = 10;
// allocate memory
numbers = malloc( N * sizeof(int));
// set values
int i;
for(i=0;i<N;i++){
numbers[i] = i+3;
}
// display values
for(i=0;i<N;i++){
printf("%d ",numbers[i]);
}
printf("\n");
// free memory
free(numbers);
return 0;
}
上面的代码生成以下结果。

注意
另一个示例,我们还定义了具有多维的动态数组。
例如,我们创建二维动态数组。
#include <stdio.h>
#include <stdlib.h>
int main(int argc, const char* argv[]) {
// define dynamic array of pointer
int **matrix; // two dimensional array pointer
// a number of array
int M = 3;
int N = 5;
// allocate memory
matrix = malloc( M * sizeof(int*));
// set values
int i,j;
for(i=0;i<M;i++){
matrix[i] = malloc( N * sizeof(int));
for(j=0;j<N;j++){
matrix[i][j] = i + j;
}
}
// display values
for(i=0;i<M;i++){
for(j=0;j<N;j++){
printf("%d ",matrix[i][j]);
}
printf("\n");
}
// free memory
free(matrix);
return 0;
}
上面的代码生成以下结果。
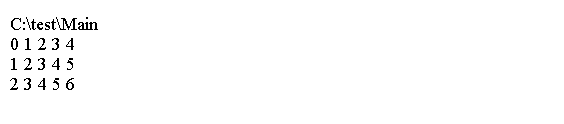
使用calloc()函数的内存分配
在stdlib.h头中声明的 calloc()
函数。
它提供了比 malloc()
的几个优势。
它将内存分配为给定大小的元素数。
它初始化分配的内存,使所有字节为零。
calloc()函数需要两个参数值:数据项的数量和每个数据项的大小。
两个参数都是 size_t
类型。
分配的区域的地址返回类型void *。
这里是如何使用 calloc()
为int类型的75个元素的数组分配内存:
int *pNumber = (int*) calloc(75, sizeof(int));
如果不可能分配所请求的内存,则返回值将为NULL。
你可以让编译器负责提供转换:
int *pNumber = calloc(75, sizeof(int));
例子
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
int main(void)
{
unsigned long long *pPrimes = NULL; // Pointer to primes storage area
int total = 5; // Number of primes required
// Allocate sufficient memory to store the number of primes required
pPrimes = calloc((size_t)total, sizeof(unsigned long long));
if (pPrimes == NULL) {
printf("Not enough memory. It"s the end I"m afraid.\n");
return 1;
}
free(pPrimes); // Release the heap memory . . .
pPrimes = NULL; // . . . and reset the pointer
return 0;
}
上面的代码生成以下结果。

扩展动态分配的内存
realloc()函数使您能够重用或扩展内存您先前使用malloc()或calloc()(或realloc())分配。
realloc()函数需要两个参数值:一个包含先前地址的指针通过调用malloc(),calloc()或realloc()返回,以及新内存的大小(以字节为单位)。
例3
由malloc()获取的各个内存段可以像数组成员一样处理;这些内存段可以用索引引用。
#include <stdio.h>
#include <stdlib.h>
main()
{
int *numbers;
int x;
numbers = (int *) malloc(5 * sizeof(int));
if ( numbers == NULL )
return; // return if malloc is not successful
numbers[0] = 100;
numbers[1] = 200;
numbers[2] = 300;
numbers[3] = 400;
numbers[4] = 500;
printf("\nIndividual memory segments initialized to:\n");
for ( x = 0; x < 5; x++ )
printf("numbers[%d] = %d\n", x, numbers[x]);
}
上面的代码生成以下结果。
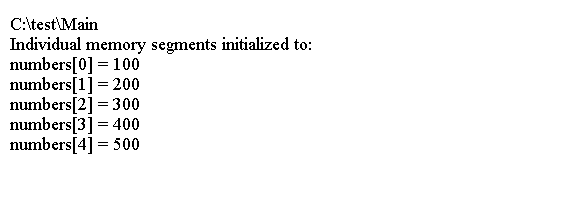
例4
以下代码显示了扩展的连续内存和realloc()的结果。
#include<stdio.h>
#include<stdlib.h>
main()
{
int *number;
int *newNumber;
int x;
number = malloc(sizeof(int) * 5);
if ( number == NULL ) {
printf("\nOut of memory!\n");
return;
} // end if
printf("\nOriginal memory:\n");
for ( x = 0; x < 5; x++ ) {
number[x] = x * 100;
printf("number[%d] = %d\n", x, number[x]);
} // end for loop
newNumber = realloc(number, 10 * sizeof(int));
if ( newNumber == NULL ) {
printf("\nOut of memory!\n");
return;
}
else
number = newNumber;
//intialize new memory only
for ( x = 5; x < 10; x++ )
number[x] = x * 100;
printf("\nExpanded memory:\n");
for ( x = 0; x < 10; x++ )
printf("number[%d] = %d\n", x, number[x]);
//free memory
free(number);
}
上面的代码生成以下结果。
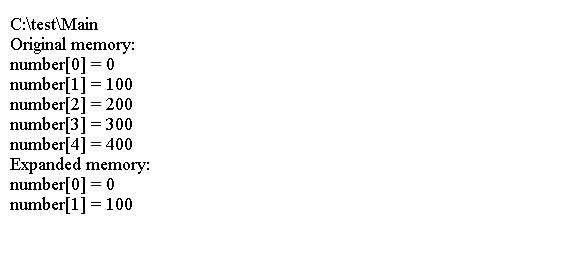
更多建议: