C 函数指针
学习C - C函数指针
声明一个函数的指针
下面的代码展示了如何声明一个指向一个函数的指针。
int (*pfunction) (int);
上面的代码声明一个变量,它是一个指向函数的指针。
这个语句只是定义了指针变量。
指针的名称是 function
,它指向的函数一个int类型的参数和一个int返回类型。
通过函数指针调用函数
假设您定义了一个具有以下原型的函数:
int sum(int a, int b);
该函数有两个类型为int的参数,返回类型为int。我们可以为它定义一个函数指针,如下所示。
int (*pfun)(int, int) = sum;
这声明一个名为pfun的函数指针。
它可以存储具有两个参数的函数的地址类型int和类型int的返回值。
该语句还使用函数 sum()
的地址初始化 pfun
。
你可以通过函数指针调用sum(),如下所示:
int result = pfun(4, 5);
这个语句通过pfun指针调用sum()函数参数值为4和5。
我们可以像函数名一样使用函数指针名称。
假设您定义了另一个具有以下原型的函数:
int divide(int a, int b);
您可以使用以下语句存储pfun中的divide()的地址:
pfun = divide;
使用包含divide()的地址的 pfun
,您可以通过指针调用divide():
result = pfun(5, 12);
以下代码具有三个具有相同参数的函数并返回类型并使用指向函数的指针来调用它们。
#include <stdio.h>
//from w ww . ja v a 2 s . co m
// Function prototypes
int sum(int, int);
int product(int, int);
int difference(int, int);
int main(void) {
int a = 10;
int b = 5;
int result = 0;
int (*pfun)(int, int); // Function pointer declaration
pfun = sum; // Points to function sum()
result = pfun(a, b); // Call sum() through pointer
printf("pfun = sum result = %2d\n", result);
pfun = product; // Points to function product()
result = pfun(a, b); // Call product() through pointer
printf("pfun = product result = %2d\n", result);
pfun = difference; // Points to function difference()
result = pfun(a, b); // Call difference() through pointer
printf("pfun = difference result = %2d\n", result);
return 0;
}
int sum(int x, int y) {
return x + y;
}
int product(int x, int y)
{
return x * y;
}
int difference(int x, int y)
{
return x - y;
}
上面的代码生成以下结果。
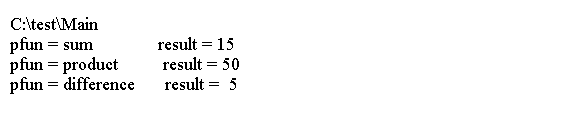
函数的指针数组
您可以创建一个指向函数的指针数组。
要声明一个函数指针数组,将数组维度放在函数指针数组名称后面。
int (*pfunctions[10]) (int);
这声明一个数组,函数,有十个元素。
此数组中的每个元素都可以存储a的地址函数,返回类型为int,参数类型为int。
下面的代码显示了如何使用指针数组到功能。
#include <stdio.h>
//from w w w . j av a 2 s .com
// Function prototypes
int sum(int, int);
int product(int, int);
int difference(int, int);
int main(void) {
int a = 10;
int b = 5;
int result = 0;
int (*pfun[3])(int, int); // Function pointer array declaration
// Initialize pointers
pfun[0] = sum;
pfun[1] = product;
pfun[2] = difference;
// Execute each function pointed to
for(int i = 0 ; i < 3 ; ++i) {
result = pfun[i](a, b); // Call the function through a pointer
printf("result = %2d\n", result);
}
// Call all three functions through pointers in an expression
result = pfun[1](pfun[0](a, b), pfun[2](a, b));
printf("The product of the sum and the difference = %2d\n", result);
return 0;
}
int sum(int x, int y) {
return x + y;
}
int product(int x, int y)
{
return x * y;
}
int difference(int x, int y)
{
return x - y;
}
上面的代码生成以下结果。
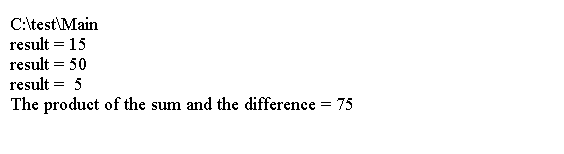
指针作为参数
您可以使用函数的指针作为函数的参数。
这样我们可以调用不同的函数这取决于指针指向哪个函数。
#include <stdio.h>
/*from w ww . j av a 2 s .c om*/
// Function prototypes
int sum(int,int);
int product(int,int);
int difference(int,int);
int any_function(int(*pfun)(int, int), int x, int y);
int main(void) {
int a = 10;
int b = 5;
int result = 0;
int (*pf)(int, int) = sum; // Pointer to function
// Passing a pointer to a function
result = any_function(pf, a, b);
printf("result = %2d\n", result );
// Passing the address of a function
result = any_function(product,a, b);
printf("result = %2d\n", result );
printf("result = %2d\n", any_function(difference, a, b));
return 0;
}
// Definition of a function to call a function
int any_function(int(*pfun)(int, int), int x, int y) {
return pfun(x, y);
}
int sum(int x, int y) {
return x + y;
}
int product(int x, int y) {
return x * y;
}
int difference(int x, int y) {
return x - y;
}
上面的代码生成以下结果。
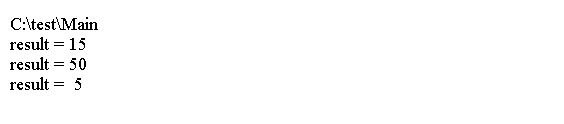
更多建议: