Spring教程 - Spring Bean初始化和销毁
Spring教程 - Spring Bean初始化和销毁
我们可以使用Spring InitializingBean和DisposableBean以对bean初始化和销毁执行操作。
对于来自Spring的Bean实现的InitializingBean,Spring IoC容器将运行 afterPropertiesSet()
方法所有的bean属性都已设置。
对于来自Spring的Bean实现的DisposableBean,它会调用destroy()在Spring容器释放bean之后。
Java Bean
这里是一个例子,显示如何使用InitializingBean和DisposableBean。
在下面的代码中定义了一个PrinterHelper bean,它实现了两者InitializingBean和DisposableBean接口。
它还有两个方法一个是 afterPropertiesSet()
方法和另一个是 destroy()
方法。
package com.www.w3cschool.cnmon; import org.springframework.beans.factory.DisposableBean; import org.springframework.beans.factory.InitializingBean; public class PrinterHelper implements InitializingBean, DisposableBean { String message; public String getMessage() { return message; } public void setMessage(String message) { this.message = message; } public void afterPropertiesSet() throws Exception { System.out.println("Init method after properties are set : " + message); } public void destroy() throws Exception { System.out.println("Spring Container is destroy! JavaBean clean up"); } @Override public String toString() { return message ; } }
XML配置文件
这里是Spring-Customer.xml for Java bean配置。
<beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-2.5.xsd"> <bean id="printerService" class="com.www.w3cschool.cnmon.PrinterHelper"> <property name="message" value="i"m property message" /> </bean> </beans>
主要方法
这里是代码显示如何运行上面的代码。
ConfigurableApplicationContext.close()调用将关闭应用程序上下文,它会调用destroy()方法。
package com.www.w3cschool.cnmon; import org.springframework.context.ConfigurableApplicationContext; import org.springframework.context.support.ClassPathXmlApplicationContext; public class App { public static void main( String[] args ) { ConfigurableApplicationContext context = new ClassPathXmlApplicationContext(new String[] {"SpringBeans.xml"}); PrinterHelper cust = (PrinterHelper)context.getBean("printerService"); System.out.println(cust); context.close(); } }
上面的代码生成以下结果。
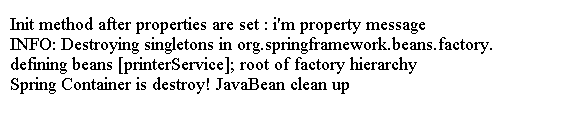
从输出我们可以看到 afterPropertiesSet()
方法被调用,之后的message属性设置和 destroy()
方法是在context.close()之后调用的;
Download Java2s_Spring_Init_Destroy.zip
Spring init方法和destroy方法
下面的代码显示了调用自定义方法的另一种方法在Java Bean中初始化和销毁Java Bean时。
我们可以使用init-method和destroy-method属性在bean配置文件中标记init方法和destroy方法在Java Bean中。
在初始化期间调用init-methoddestroy方法在销毁期间被调用。
下面的代码显示了如何在Java Bean中添加方法来分别做init和destroy。
package com.www.w3cschool.cnmon; public class PrinterHelper { String message; public String getMessage() { return message; } public void setMessage(String message) { this.message = message; } public void initIt() throws Exception { System.out.println("Init method after properties are set : " + message); } public void cleanUp() throws Exception { System.out.println("Spring Container is destroy! Customer clean up"); } @Override public String toString() { return message; } }
在下面的xml配置文件中使用 init-method
和 destroy-method
属性进行标记init方法和destroy方法。
<beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-2.5.xsd"> <bean id="printerService" class="com.www.w3cschool.cnmon.PrinterHelper" init-method="initIt" destroy-method="cleanUp"> <property name="message" value="i"m property message" /> </bean> </beans>
Download Java2s_Spring_init-method.zip
Spring init方法和destroy方法...
Spring还支持标记为Java Bean的@PostConstruct和@PreDestroy注释。
我们使用这两个注释来标记init-method和destroy-method。
@PostConstruct和@PreDestroy注释来自J2ee common-annotations.jar。
在下面的代码中,我们使用@PostConstruct和@PreDestroy注释以标记PrinterHelper类。
package com.java2s.customer.services; import javax.annotation.PostConstruct; import javax.annotation.PreDestroy; public class PrinterHelper { String message; public String getMessage() { return message; } public void setMessage(String message) { this.message = message; } @PostConstruct public void initIt() throws Exception { System.out.println("Init method after properties are set : " + message); } @PreDestroy public void cleanUp() throws Exception { System.out.println("Spring Container is destroy! Customer clean up"); } }
在下面的代码中,我们使用@PostConstruct和@PreDestroy注释以标记PrinterHelper类。...
以下xml配置文件调用CommonAnnotationBeanPostProcessorJava Bean。
<beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-2.5.xsd"> <bean class="org.springframework.context.annotation.CommonAnnotationBeanPostProcessor" /> <bean id="printerService" class="com.www.w3cschool.cnmon.PrinterHelper"> <property name="message" value="i"m property message" /> </bean> </beans>
以下xml配置文件调用CommonAnnotationBeanPostProcessorJava Bean。...
<beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:context="http://www.springframework.org/schema/context" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-2.5.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-2.5.xsd"> <context:annotation-config /> <bean id="printerService" class="com.www.w3cschool.cnmon.PrinterHelper"> <property name="message" value="i"m property message" /> </bean> </beans>
更多建议: